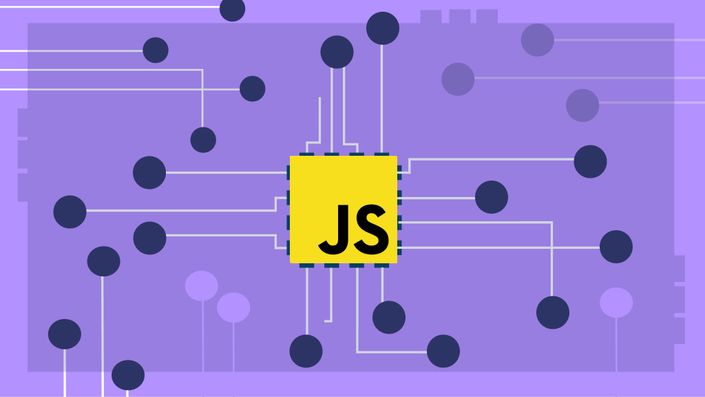
JavaScript Data Structures - The Fundamentals
Learn all the core basics about JS data structures - both built-in ones like Maps, Sets or Arrays as well as custom ones like Linked Lists, Trees, Graphs etc.
Watch Promo Enroll in Course
When working with JavaScript, you'll work with data structures all the time.
That includes built-in ones like Arrays, Objects, Maps or Sets but - especially if you dive deeper into JavaScript - also custom data structures like Linked Lists, Trees or Graphs.
This course covers it all!
We'll recap the basic built-in data structures to understand how they work and when to use them, before we then explore important custom data structures.
In detail, this course covers the following structures:
- Arrays (built-in)
- Objects (built-in)
- Sets (built-in)
- Maps (built-in)
- Linked Lists (custom)
- Stacks (custom)
- Queues (custom)
- Hash Tables (custom)
- Basic Trees (custom)
- Binary Search Trees (BST, custom)
- AVL Trees (custom)
- Priority Queues (custom)
- Heaps (custom)
- Graphs (custom
You will learn how those different data structures work, when they might be helpful, how to build them and of course we'll also explore their time complexity (Big O notation)!
You'll get both the theory as well as practical examples to ensure that you fully understand all those key structures and you're able to work with them on your own after you finished this course - no matter if you need them for your next project or simply to ace an upcoming job interview!
To get the most out of this course, you should ensure that you have a basic understanding of JavaScript algorithms and that you especially understand the concept of time complexity and "Big O" notation. In case you're missing those prerequisites right now, you can explore our Algorithms course!
9+
hours
90+
lessons
Course Curriculum
-
PreviewWelcome to the Course! (1:11)
-
PreviewWhat are "Data Structures"? (4:58)
-
PreviewCourse Resources
-
PreviewArrays: Recap (8:57)
-
PreviewSets: Recap (5:46)
-
PreviewArrays vs Sets (1:34)
-
PreviewObjects: Recap (7:05)
-
PreviewMaps: Recap (7:37)
-
PreviewObjects vs Maps (1:43)
-
PreviewWeakSet and WeakMap (2:16)
-
PreviewLinked Lists (A First Custom Data Structures) (2:46)
-
PreviewStarting with Linked Lists and "append" (13:19)
-
PreviewOutputting the Linked List (4:58)
-
PreviewPrepend to Linked Lists (4:09)
-
PreviewDeleting Nodes (10:31)
-
Preview"find" and "insertAfter" (6:56)
-
PreviewLinked Lists: Why and Time Complexity (5:28)
-
PreviewLinked Lists vs Arrays (7:06)
-
PreviewModule Resources
-
StartThe Academind Pro Referral Program
-
StartModule Introduction (0:55)
-
StartWhat are "Lists" and "Tables"? (4:22)
-
StartBuilt-in Lists and Tables (3:42)
-
StartIntroducing "Stacks" (5:51)
-
StartBuilding a "Stack" Data Structure (7:01)
-
StartConsidering a Linked List Stack (2:41)
-
StartStacks with Linked Lists (9:12)
-
StartStacks: Time Complexity (4:50)
-
StartIntroducing Queues (3:24)
-
StartBuilding a Queue (4:34)
-
StartLinked Lists & Queues (5:09)
-
StartQueue Time Complexity (4:45)
-
StartIntroducing Hash Tables (4:18)
-
StartWhy we need Tables (9:54)
-
StartBuilding a Basic Hash Table (12:20)
-
StartUnderstanding Hash Collisions (4:58)
-
StartSolving Collisions with Chaining (11:51)
-
StartSolving Collisions with Open Addressing (10:42)
-
StartHash Tables: Time Complexity (7:04)
-
StartHash Tables: Summary (4:46)
-
StartModule Resources
-
StartModule Introduction (1:35)
-
StartWhat are "Trees"? (3:28)
-
StartCore Terminology (8:58)
-
StartAn Example Tree (2:43)
-
StartA First Tree in Code (11:16)
-
StartA Better Filesystem Tree (14:10)
-
StartRemoving Tree Nodes (7:06)
-
StartDescribing Our Tree (6:49)
-
StartTrees: Time Complexity (4:42)
-
StartDepth-first vs Breadth-first Search (2:21)
-
StartImplementing Depth-first Search (6:01)
-
StartImplementing Breadth-first Search (3:27)
-
StartDepth-first Search vs Breadth-first Search (5:06)
-
StartIntroducing Binary Search Trees (BST) (3:34)
-
StartAdding Values to BST (6:27)
-
StartFinding Values in BST (10:13)
-
StartRemoving Leaf Nodes in BST (7:46)
-
StartRemoving "One-Child" Nodes in BST (5:12)
-
StartRemoving "Multi-Child" Nodes in BST (12:09)
-
StartBST Time Complexity (3:38)
-
StartIntroducing AVL Trees (3:08)
-
StartBalancing Trees with Rotations (4:05)
-
StartUnderstanding Balance Factors (2:59)
-
StartAdding Depth and Balance Factors (6:25)
-
StartFinding the Right Rotation (8:07)
-
StartImplementing Left Rotation (9:50)
-
StartImplementing Right Rotation (3:21)
-
StartImplementing Left-Right Rotation (8:10)
-
StartImplementing Right-Left Rotation (3:28)
-
StartTesting the AVL Tree (3:06)
-
StartAVL vs BST (1:05)
-
StartIntroducing Tries (3:35)
-
StartBuilding a Trie (12:01)
-
StartFinding Nodes in a Trie (3:24)
-
StartDeleting Keys (3:57)
-
StartTries: Time Complexity and Comparison to Hash Tables (5:41)
-
StartModule Resources
Course Prerequisites
Algorithm basics are required - especially knowledge about time complexity (e.g. take our Algorithms course)
All pre-requisites are covered by courses in our "Academind Pro" Membership.
30 Day Money Back Guarantee - No Questions Asked!
Our courses helped thousands of students learn something new and improve their lives.
We're so convinced by our course quality that we guarantee your success and provide a full refund within the first 30 days if you're not happy with the course.
Your Instructor

As a self-taught professional I really know the hard parts and the difficult topics when learning new or improving on already-known languages. This background and experience enable me to focus on the most relevant key concepts and topics. My track record of many 5-star rated courses, more than 2,500,000 students worldwide as well as a successful YouTube channel with 900.000 subscribers is the best proof for that.
The most rewarding experience for me is to see how people find new, better jobs, build awesome web applications, work on amazing projects or simply enjoy their hobby with the help of my content.
That's why, together with Manuel Lorenz, I founded Academind to offer the best possible learning experience and to share the pleasure of learning with our students.
Frequently Asked Questions
Join 9652 happy students!
Single-Course License
Full access to "JavaScript Data Structures - The Fundamentals
$89
Access to this course only.
This is a one-time payment that grants access to this course only, not to any other courses.
Academind Pro Membership
Unlimited access to this and all other current & future courses!